In this chapter, you will learn:
- What is Html.BeginForm?
- How to Create Html.BeginForm
- How to Pass Form Data to Controller
- Complete Programming Example
WHAT IS HTML.BEGINFORM?
Html.BeginForm is the Html Helper Extension Method that is used for creating and rendering the form in HTML. This method makes your job easier in creating form. Here, is the method to create a form using Html.BeginForm extension method in ASP.NET MVC5.
Html.BeginForm("ActionMethod", "ControllerName","Get⁄Post Method")
Generally, 3 parameters are used when creating Html.BeginForm
ActionMethod – It defines which action method is look for when submit button is clicked.
ControllerName – It defines, which controller keeps the defined action method.
Get/Post Method – it defines the method you want to use to send data from form to controller.
PROGRAMMING EXAMPLE:
- namespace HtmlHelperDemo.Models
- {
- public class UserModel
- {
- public string UserName { get; set; }
- public int Age { get; set; }
- public string City { get; set; }
- }
- }
- @using HtmlHelperDemo.Models
- @model UserModel
- <h1>Html.DisplayFor Example</h1>
- @using (Html.BeginForm("Index", "Home", FormMethod.Post))
- {
- <span>Enter Your Name:</span> @Html.TextBoxFor(m => m.UserName)<br />
- <span>Enter Your Age: </span> @Html.TextBoxFor(m => m.Age)<br />
- <span>Enter Your City: </span>@Html.TextBoxFor(m => m.City)<br />
- <input id = "Submit" type = "submit" value = "submit" />
- }
- <hr />
- <strong>User Name: </strong> @Html.DisplayFor(m => m.UserName)<br />
- <strong>User Age: </strong> @Html.DisplayFor(m => m.Age)<br />
- <strong>User City: </strong> @Html.DisplayFor(m => m.City)<br />
- namespace HtmlHelperDemo.Controllers
- {
- public class HomeController : Controller
- {
- public ActionResult Index()
- {
- return View();
- }
- [HttpPost]
- public ActionResult Index(UserModel um)
- {
- return View(um);
- }
- }
- }
OUTPUT
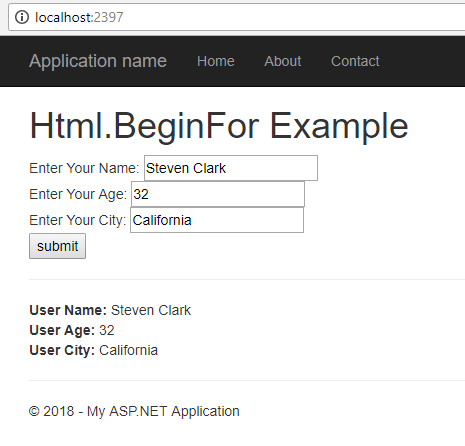
SUMMARY:
However, there is a lot thing to learn in WebForms but this chapter focuses on simple understanding on how Html.BeginForm can be created. I added the simple but complete programming example that will surely help you to create a form using Html.BeginForm Extension Method. However, I have also written some advanced topic on WebForms and if you want to learn more about this, please visit the following article.
https://www.completecsharptutorial.com/asp-net-mvc5/html-beginform-example-tutorial-aspnet-mvc5.php